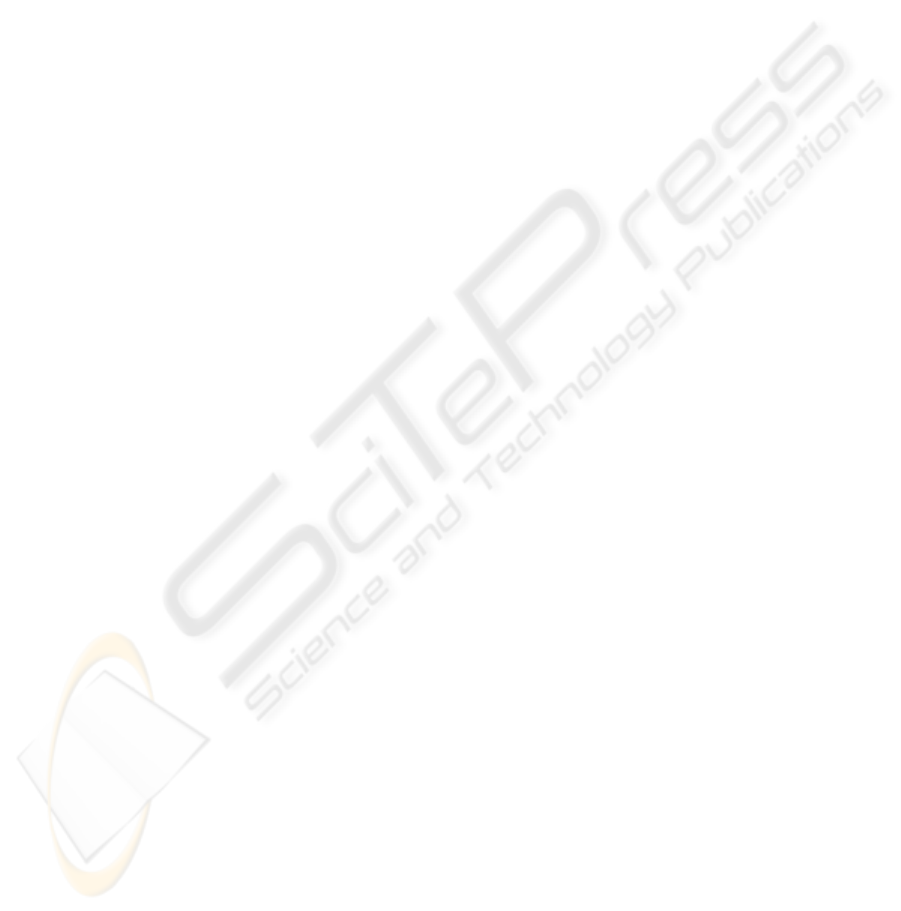
lowing. A problem is recursively divided into sub-
problems and lower bounds for the optimal solution
of each subproblem are computed. If a solution of
a (sub)problem is found, it is also a solution of the
overall problem. Then, all other subproblems can
be discarded, whose corresponding lower bounds are
greater than the value of the solution. Subproblems
with smaller lower bounds still have to considered re-
cursively.
Only little related work on algorithmic skeletons
for branch & bound can be found in the literature
(E. Alba, 2002; F. Almeida, 2001; I. Dorta, 2003;
Hofstedt, 1998). However, in the corresponding liter-
ature there is no discussion of different designs. The
MaLLBa implementation is based on a master/worker
scheme and it uses a central queue (rather than a heap)
for storing problems. The master distributes problems
to workers and receives their solutions and generated
subproblems. On a shared memory machine this ap-
proach can work well. We will show in the sequel
that a master/worker approach is less suited to handle
branch & bound problems on distributed memory ma-
chines. In a previous version of the Muesli skeleton
library, a branch & bound skeleton with a centralized
work pool has bee used, too (H. Kuchen, 2002). Hof-
stedt outlines a B&B skeleton with a distributed work
pool. Here, work is only shared, if a local work pool
is empty. Thus, worthwhile problems are not propa-
gated quickly and their investigation is concentrated
on a few workers only.
The rest of this paper is structured as follows. In
Section 2, we recall, how branch & bound algorithms
can be used to solve optimization problems. In Sec-
tion 3, we introduce different designs of branch &
bound skeletons in the framework of the skeleton li-
brary Muesli (Kuchen, 2002; Kuchen, 2004; Kuchen,
2006). After describing the simple centralized design
considered in (H. Kuchen, 2002), we will focus on
a design with a distributed work pool. Section 4 con-
tains experimental results demonstrating the strengths
and weaknesses of the different designs. In Section 5,
we conclude and point out future work.
2 BRANCH & BOUND
Branch & bound algorithms are general methods used
for solving difficult combinatorial optimization prob-
lems. In this section, we illustrate the main prin-
ciples of branch & bound algorithms using the 8-
puzzle, a simplified version of the well-known 15-
puzzle (Quinn, 1994), as example. A branch & bound
algorithm searches the complete solution space of a
given problem for the best solution. Due to the ex-
ponentially increasing number of feasible solutions,
their explicit enumeration is often impossible in prac-
tice. However, the knowledge about the currently best
solution, which is called incumbent, and the use of
bounds for the function to be optimized enables the
algorithm to search parts of the solution space only
implicitly. During the solution process, a pool of yet
unexplored subsets of the solution space, called the
work pool, describes the current status of the search.
Initially there is only one subset, namely the com-
plete solution space, and the best solution found so
far is infinity. The unexplored subsets are repre-
sented as nodes in a dynamically generated search
tree, which initially only contains the root, and each
iteration of the branch & bound algorithm processes
one such node. This tree is called the state-space tree.
Each node in the state-space tree has associated data,
called its description, which can be used to determine,
whether it represents a solution and whether it has any
successors. A branch & bound problem is solved by
applying a small set of basic rules. While the signa-
ture of these rules is always the same, the concrete
formulation of the rules is problem dependent. Start-
ing from a given initial problem, subproblems with
pairwise disjoint state spaces are generated using an
appropriate branching rule. A generated subproblem
can be estimated applying a bounding rule. Using a
selection rule, the subproblem to be branched from
next is chosen from the work pool. Last but not least
subproblems with non-optimal or inadmissible solu-
tions can be eliminated during the computation using
an elimination rule. The sequence of the application
of these rules may vary according to the strategy cho-
sen for selecting the next node to process (J. Clausen,
1999). As an example of the branch and bound tech-
nique, consider the 8-puzzle (Quinn, 1994). Figure 1
illustrates the goal state of the 8-puzzle and the first
three levels of the state-space tree.
The 8-puzzle consists of eight tiles, numbered 1
through 8, arranged on a 3 × 3 board. Eight positions
on the board contain exactly one tile and the remain-
ing position is empty. The objective of the puzzle is
to repeatedly fill the hole with a tile adjacent to it in
horizontal or vertical direction, until the tiles are in
row major order. The aim is to solve the puzzle in the
least number of moves.
The branching rule describes, how to split a prob-
lem represented by a given initial board into subprob-
lems represented by the boards resulting after all valid
moves. A minimum number of tile moves needed to
solve the puzzle can be estimated by adding the num-
ber of tile moves made so far to the Manhattan dis-
tance between the current position of each tile and its
goal position. The computation of this lower bound is
described by the bounding rule.
The state-space tree represents all possible boards
that can be reached from the initial board. One way
to solve this puzzle is to pursue a breadth first search
or a depth first search of the state-space tree until the
ICSOFT 2006 - INTERNATIONAL CONFERENCE ON SOFTWARE AND DATA TECHNOLOGIES
292