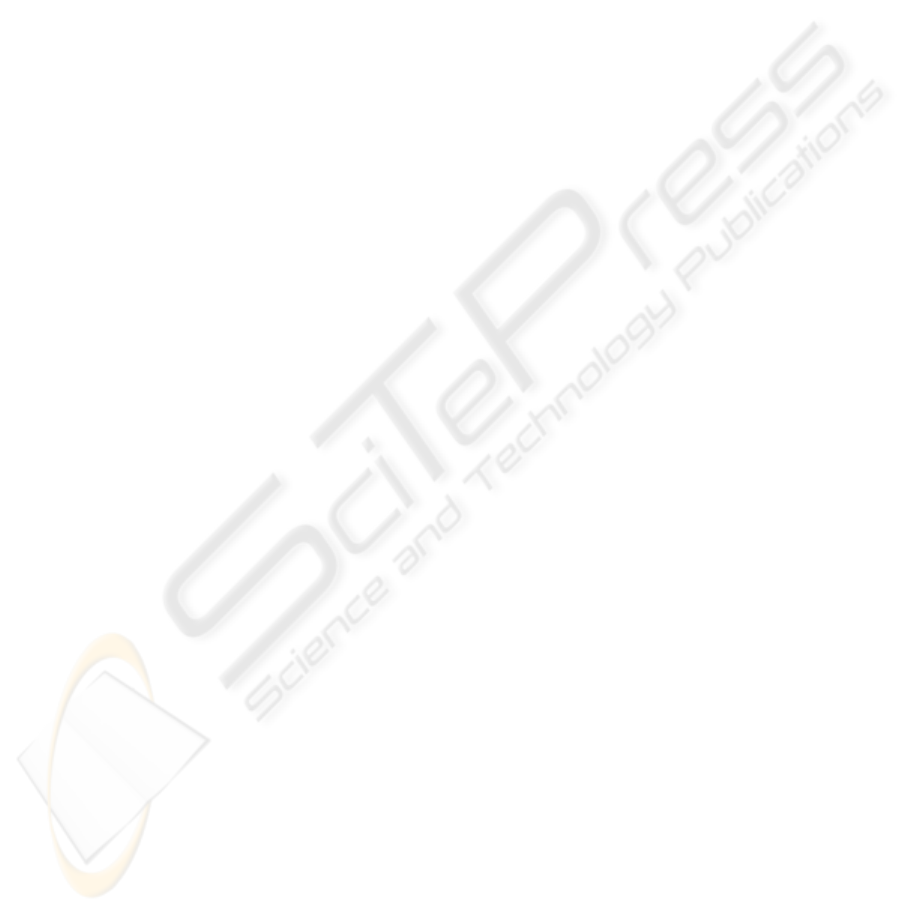
7. Object Management Group. Unified Modeling Language (UML) Specification, Version 1.4,
September 2001 (Version 1.3, June 1999). Available at http://www.omg.org/.
8. Perdita Stevens. “On the Interpretation of Binary Associations in the Unified Modelling
Language”, Journal on Software and Systems Modeling, 1(1):68-79 (2002). A preliminar
version in: Perdita Stevens. "On Associations in the Unified Modeling Language". The
Fourth International Conference on the Unified Modeling Language, UML'2001, October
1-5, 2001, Toronto, Ontario, Canada. Published in Lecture Notesin Computer Science 285,
Springer 2001, pp. 361-375.
9. Gonzalo Génova. "Semantics of Navigability in UML Associations". Technical Report
UC3M-TR-CS-2001-06, Computer Science Department, Carlos III University of Madrid,
November 2001, pp. 233-251.
10. Gonzalo Génova, Juan Llorens, Paloma Martínez. “The Meaning of Multiplicity of N-ary
Associations in UML”, Software and Systems Modeling, 1(2): 86-97, 2002. A preliminary
version in: Gonzalo Génova, Juan Llorens, Paloma Martínez. “Semantics of the Minimum
Multiplicity in Ternary Associations in UML”. The 4th International Conference on the
Unified Modeling Language-UML'2001, October 1-5 2001, Toronto, Ontario, Canada.
Published in Lecture Notes in Computer Science 285, Springer 2001, pp. 329-341.
11. Gonzalo Génova, Juan Llorens, Vicente Palacios. "Sending Messages in UML", Journal of
Object Technology, vol.2, no.1, Jan-Feb 2003, pp. 99- 115,
http://www.jot.fm/issues/issue_2003_01/article3.
12. Il-Yeol Song, Mary Evans, E.K. Park. "A Comparative Analysis of Entity- Relationship
Diagrams", Journal of Computer and Software Engineering, 3(4):427-459 (1995).
13. William Harrison, Charles Barton, Mukund Raghavachari. "Mapping UML Designs to
Java". The 15th Annual ACM Conference on Object-Oriented Programming, Systems, Lan-
guages, and Applications-OOPSLA’2000, October 15-19 2000, Minneapolis, Minnesota,
United States. ACM SIGPLAN Notices, 35(10): 178-187. ACM Press, New York, NY,
USA.
14. Guy Genilloud. "Informal UML 1.3 - Remarks, Questions, and some Answers". UML
Semantics FAQ Workshop (held at ECOOP'99), Lisbon, Portugal, June 12th 1999.
15. The Fujaba CASE Tool, University of Paderborn, http://www.fujaba.de/.
16. Java Community Process. Java Metadata Interface (JMI) Specification, Version 1.0, June
2002. Available at http://www.jcp.org/.
53